Hello everyone,
I'm looking for some tips, to enhance the code listed here to simplify with less code the "Select case'.
This code is for a PIC18F25K22 using PortC as a 64 switches matrix scan and PortB as the receiver and decoder and PortA to send the data.
Thanks for any comments.
Code:
' PIC18F25K22 64 Keys Encoder
' File :"D:\PIC\PBP3\18F25K22\64 Keys Encoder\64 Keys Encoder.pbp"
' Date : Feb 13 2025
'
' WPUB : p152 PORTB only
' PORTA : Flags
' PORTB : Row Input
' PORTC : Column Out
;----[18F25K22 Hardware Configuration]------------------------------------------
#CONFIG
CONFIG FOSC = INTIO67 ; Internal oscillator block
CONFIG PLLCFG = ON ; Oscillator used directly
CONFIG PRICLKEN = OFF ; Primary clock can be disabled by software
CONFIG FCMEN = OFF ; Fail-Safe Clock Monitor disabled
CONFIG IESO = OFF ; Oscillator Switchover mode disabled
CONFIG PWRTEN = OFF ; Power up timer disabled
CONFIG BOREN = SBORDIS ; Brown-out Reset enabled in hardware only (SBOREN is disabled)
CONFIG BORV = 190 ; VBOR set to 1.90 V nominal
CONFIG WDTEN = ON ; WDT is always enabled. SWDTEN bit has no effect
CONFIG WDTPS = 32768 ; 1:32768
CONFIG CCP2MX = PORTC1 ; CCP2 input/output is multiplexed with RC1
CONFIG PBADEN = OFF ; PORTB<5:0> pins are configured as digital I/O on Reset
CONFIG CCP3MX = PORTB5 ; P3A/CCP3 input/output is multiplexed with RB5
CONFIG HFOFST = ON ; HFINTOSC output and ready status are not delayed by the oscillator stable status
CONFIG T3CMX = PORTC0 ; T3CKI is on RC0
CONFIG P2BMX = PORTB5 ; P2B is on RB5
CONFIG MCLRE = INTMCLR ; MCLR pin enabled, RE3 input pin disabled
CONFIG STVREN = ON ; Stack full/underflow will cause Reset
CONFIG LVP = OFF ; Single-Supply ICSP disabled
CONFIG XINST = OFF ; Instruction set extension and Indexed Addressing mode disabled (Legacy mode)
CONFIG DEBUG = OFF ; Disabled
CONFIG CP0 = OFF ; Block 0 (000800-001FFFh) not code-protected
CONFIG CP1 = OFF ; Block 1 (002000-003FFFh) not code-protected
CONFIG CP2 = OFF ; Block 2 (004000-005FFFh) not code-protected
CONFIG CP3 = OFF ; Block 3 (006000-007FFFh) not code-protected
CONFIG CPB = OFF ; Boot block (000000-0007FFh) not code-protected
CONFIG CPD = OFF ; Data EEPROM not code-protected
CONFIG WRT0 = OFF ; Block 0 (000800-001FFFh) not write-protected
CONFIG WRT1 = OFF ; Block 1 (002000-003FFFh) not write-protected
CONFIG WRT2 = OFF ; Block 2 (004000-005FFFh) not write-protected
CONFIG WRT3 = OFF ; Block 3 (006000-007FFFh) not write-protected
CONFIG WRTC = OFF ; Configuration registers (300000-3000FFh) not write-protected
CONFIG WRTB = OFF ; Boot Block (000000-0007FFh) not write-protected
CONFIG WRTD = OFF ; Data EEPROM not write-protected
CONFIG EBTR0 = OFF ; Block 0 (000800-001FFFh) not protected from table reads executed in other blocks
CONFIG EBTR1 = OFF ; Block 1 (002000-003FFFh) not protected from table reads executed in other blocks
CONFIG EBTR2 = OFF ; Block 2 (004000-005FFFh) not protected from table reads executed in other blocks
CONFIG EBTR3 = OFF ; Block 3 (006000-007FFFh) not protected from table reads executed in other blocks
CONFIG EBTRB = OFF ; Boot Block (000000-0007FFh) not protected from table reads executed in other blocks
#ENDCONFIG
define OSC 64 ' OSC 64Mhz
ANSELA = 0 ' Set all digital
ANSELB = 0 ' Set all digital
ANSELC = 0 ' Set all digital
WPUB = $FF ' Set Weak PullUP PORTB only
'CMCON0 = 7
TRISA = 000000 ' PORTA Key Output
TRISB = 111111 ' PORTB Intput Keypad Rows in
TRISC = 000000 ' PORTC Output Keypad Column out
TRISE = 001000 ' PORTE.3 MCLRE as input '1' other output '0'
OSCCON = $70 ' Internal OSC p:30
OSCTUNE = $40 ' for 64Mhz FOSC p:35
Latch1 var byte ' Latch1 Flag
Latch1 = 0
LRShift VAR BIT ' Shift Direction Flag
LRSHIFT = 0
TTL VAR WORD 'Key val
TTL = 0
TTLadd var word ' adding to PORTC val
ttladd = 0
PORTKey var byte ' Key data
portkey = 0
Baud var word ' Serout2 Baud Rate
Baud = 84
'Baud = 16468
PORTB = 0 ' PORTB set to 0 Keypad Row
PAUSE 10
PORTC = 000001 ' Keypad Column out to Row PORTB
PORTA.0 = 1 ' serial port = 1 'avoid random data'
PORTA.1 = 0 ' Data Available
MAIN: ' Main Routine
PAUSE 1 ' needed for PORTC > PORTB Data transfert
LATB = PORTB ' Needed to use the value of PORTB
' Output Data to PORTC
IF PORTB > 0 AND Latch1 = 0 THEN
Latch1 = 1
LATC = LATB
PAUSE 5
' prevent duplicate
IF PORTC = 1 THEN
TTLADD = 0
endif
IF PORTC = 2 THEN
TTLADD = 128
endif
IF PORTC = 4 THEN
TTLADD = 384
endif
IF PORTC = 8 THEN
TTLADD = 512
endif
IF PORTC = 16 THEN
TTLADD = 640
endif
IF PORTC = 32 THEN
TTLADD = 758
endif
IF PORTC = 64 THEN
TTLADD = 896
endif
IF PORTC = 128 THEN
TTLADD = 1024
endif
' prevent duplicate
TTL = TTLADD + PORTB
' Select PORTKkey val
Select Case ttl
case 1
portkey = 1 : goto P
case 2
portkey = 2 : goto P
case 4
portkey = 3 : goto P
case 8
portkey = 4 : goto P
case 16
portkey = 5 : goto P
case 32
portkey = 6 : goto P
case 64
portkey = 7 : goto P
case 128
portkey = 8 : goto P
case 129
portkey = 9 : goto P
case 130
portkey = 10 : goto P
case 132
portkey = 11 : goto P
case 136
portkey = 12 : goto P
case 144
portkey = 13 : goto P
case 160
portkey = 14 : goto P
case 192
portkey = 15 : goto P
case 256
portkey = 16 : goto P
case 385
portkey = 17 : goto P
case 386
portkey = 18 : goto P
case 388
portkey = 19 : goto P
case 392
portkey = 20 : goto P
case 400
portkey = 21 : goto P
case 416
portkey = 22 : goto P
case 448
portkey = 23 : goto P
case 512
portkey = 24 : goto P
case 513
portkey = 25 : goto P
case 514
portkey = 26 : goto P
case 516
portkey = 27 : goto P
case 520
portkey = 28 : goto P
case 528
portkey = 29 : goto P
case 544
portkey = 30 : goto P
case 576
portkey = 31 : goto P
case 640
portkey = 32 : goto P
case 641
portkey = 33 : goto P
case 642
portkey = 34 : goto P
case 644
portkey = 35: goto P
case 648
portkey = 36 : goto P
case 656
portkey = 37 : goto P
case 672
portkey = 38 : goto P
case 704
portkey = 39 : goto P
case 768
portkey = 40 : goto P
case 759
portkey = 41 : goto P
case 760
portkey = 42 : goto P
case 762
portkey = 43 : goto P
case 766
portkey = 44 : goto P
case 774
portkey = 45 : goto P
case 790
portkey = 46 : goto P
case 822
portkey = 47 : goto P
case 886
portkey = 48 : goto P
case 897
portkey = 49 : goto P
case 898
portkey = 50 : goto P
case 900
portkey = 51 : goto P
case 904
portkey = 52 : goto P
case 912
portkey = 53 : goto P
case 928
portkey = 54 : goto P
case 960
portkey = 55 : goto P
case 1024
portkey = 56 : goto P
case 1025
portkey = 57 : goto P
case 1026
portkey = 58 : goto P
case 1028
portkey = 59 : goto P
case 1032
portkey = 60 : goto P
case 1040
portkey = 61 : goto P
case 1056
portkey = 62 : goto P
case 1088
portkey = 63 : goto P
case 1152
portkey = 64 : goto P
end select
' Select PORTKkey val
P:
pause 10
PORTA.1 = 1 ' Data Available
'SEROUT2 PORTA.0,Baud,[dec3 LATC," PORTC Bin: ", bin8 LATC,13,10] ' SW PORTC Column to PORTB Row
'SEROUT2 PORTA.0,Baud,[dec3 LATB," PORTB Bin: ", bin8 LATB," DEC TTL : ",DEC5 TTL," BIN TTL: ",BIN16 TTL,13,10] ' SW PORTB Row from PORTC Column
sEROUT2 PORTA.0,Baud,[" PORTB Dec :",dec3 LATB," PORTB Bin: ", bin8 LATB," TTL Dec : ",DEC4 TTL," TTL Bin : ",BIN16 TTL," Port Key: ",dec2 portkey," Port Key Bin : ",bin8 portkey,13,10]
ENDIF
' Wait for Key Release
WHILE PORTB > 0
WEND
' Reset Flag
latch1 = 0 ' Latch1
PORTA.1 = 0 ' Data Available
LATC = 0 ' LATC
TTL = 0
pause 50 ' Debounce pause
' Shift Direction Flag
IF PORTC = 1 THEN
lrshift = 0
endif
IF PORTC = 128 THEN
lrshift = 1
endif
' Shift PORTC
if lrshift = 0 then
PORTC = PORTC << 1 ' Shift Left
endif
if lrshift = 1 then
PORTC = PORTC >> 1 ' Shift Right
ENDIF
goto main ' restart loop
Attachment 0
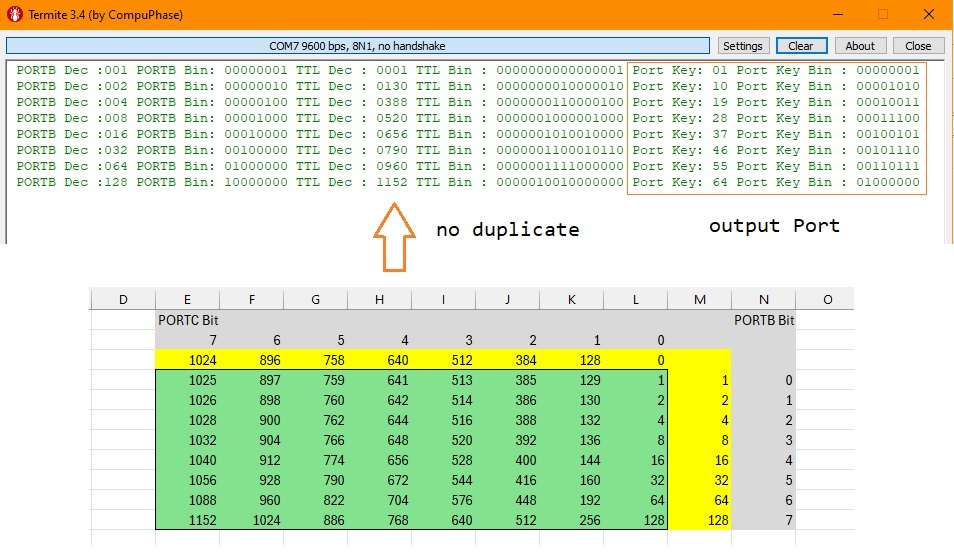
Attachment 0
Bookmarks