Hi guys,
I just did some tests here. Here's the testcode:
Code:
Leader VAR BYTE
myArray VAR BYTE[3]
Trailer VAR byte
Leader = 170
Trailer = 85
Test1:
myArray[0] = 1
myArray[1] = 127
myArray[2] = 255
PortB.5 = 0
SHIFTOUT PortB.6, PortB.7, 1, [myArray\24]
PortB.5 = 1
PauseUs 10
Test2:
myArray[0] = 255
myArray[1] = 127
myArray[2] = 1
PortB.5 = 0
SHIFTOUT PortB.6, PortB.7, 1, [myArray\24]
PortB.5 = 1
Pauseus 10
Test3:
myArray[0] = 1
myArray[1] = 127
myArray[2] = 255
PortB.5 = 0
SHIFTOUT PortB.6, PortB.7, 1, [myArray[0], myArray[1], myArray[2]]
PortB.5 = 1
Pause 100
END
The idea with the Leader and Trailer bytes was to see if the memory is alocated in the order it declared and if the shiftout would "fall into" those - turns out that's not the case in this example. Here are the captured results:
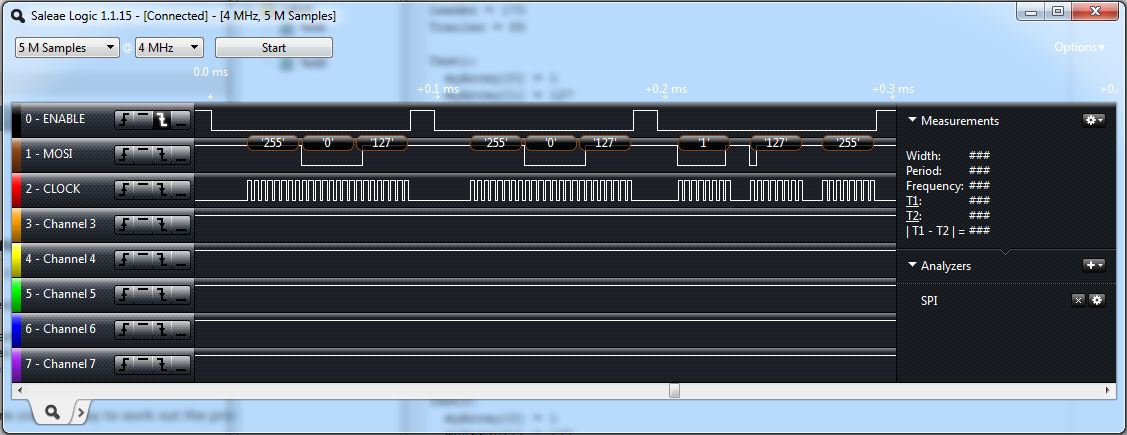
I was a bit surprised that both test 1 and test 2 gave the same results but it did.
/Henrik.
Bookmarks