Hi,
I've stared at this for most part of the day, re-written, made testcases etc etc without coming closer to the problem, I really need a fresh pair of eyes on this.
What I'm working on is a BASE64 encoder for the SMTP-engine of the W5100 project. I had it working nicely (and I can revert if this doesn't work out) but I was trying to save some RAM and ended up in this mess. Basically what I'm trying to do is copying bits from one place in to another in an array of bytes. The array is currently 256 bytes long and I'm trying to copy data from the start of it to middle - bit by bit.
Here's the current test-case code to demonstrate the problem:
Code:
BufferLength CON 256
BufferOffset CON BufferLength/2
Buffer VAR Byte[BufferLength]
ByteCount VAR BYTE
GetBit VAR WORD
PutBit VAR WORD
BitCount VAR BYTE
BufferByteIndex VAR BYTE
BufferBitIndex VAR BYTE
Temp VAR BIT
i VAR BYTE
ByteCount = 0
BitCount = 7
'Preload the Buffer array with the text 123
ArrayWrite Buffer,["123",0]
BufferByteIndex = BufferOffset
HSEROUT["BufferByteIndex: ", DEC BufferByteIndex,13]
' We're just doing three bytes for this test.
While ByteCount < 3
Buffer[BufferByteIndex] = 0 ' Clear current "out-byte" (should be 128, 129,130, 131)
HSEROUT["Buffer[", DEC BufferByteIndex, "]=", DEC Buffer[BufferByteIndex],13]
' Do it in chunks of 6 bits.
For BufferBitIndex = 5 to 0 Step -1
' Calculate where to get the bit
Getbit = ByteCount * 8 + BitCount
'Calculate where to put the bit
Putbit = BufferByteIndex * 8 + BufferBitIndex
HSEROUT["Read Byte ", #ByteCount, ".", DEC BitCount, " (GetBit=", DEC Getbit, ")"]
HSEROUT[". Write byte ", #BufferByteIndex, ".", DEC BufferBitIndex]
HSEROUT[" (PutBit=", DEC Putbit, "). Bitstate is: ", DEC Buffer.0[Getbit] ]
Temp = Buffer.0[GetBit]
HSEROUT[" Temp: ", DEC Temp,13]
'-----------------------------------------------------------------------------------
' Buffer.0[Putbit] = Temp
' HSEROUT[" Reading back: ", DEC Buffer.0[Putbit],13]
'-----------------------------------------------------------------------------------
BitCount = BitCount - 1 ' Next bit in original string
If BitCount = 255 THEN ' All bits in byte are processed
ByteCount = ByteCount + 1 ' Point to next byte
BitCount = 7 ' Start at bit 7
ENDIF
NEXT
BufferByteIndex = BufferByteIndex + 1 ' Next byte in "Output string".
Wend
' Display the content of the low end of buffer
HSEROUT["Buffer[0] to Buffer[2]: "]
For i = 0 to 2
HSEROUT[DEC Buffer[i],","]
NEXT
'Display the content in the middle of the buffer
HSEROUT [13, "Buffer [128] to [131]: "]
For i = 128 to 131
HSEROUT[DEC Buffer[i],","]
NEXT
HSEROUT [13]
Pause 100
END
Now, the output of this code looks like this: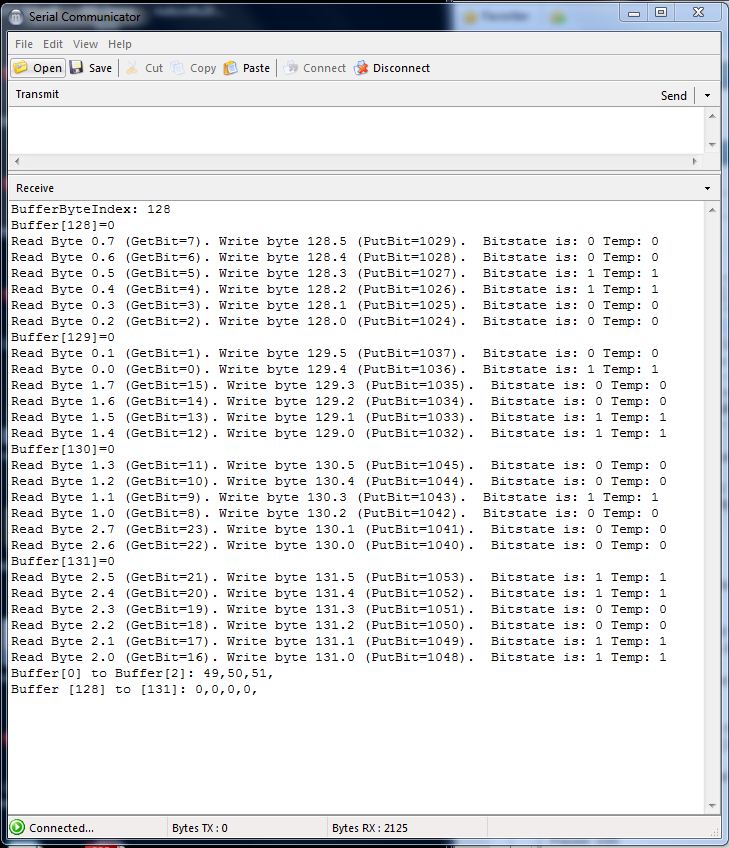
The purpose is to copy the bits from the low end of the array to the middle of it in chunks of 6bits. The 6 high bits of the first byte ends up in the 6 low bits of byte 128, the next 6bits ends up in the low end of byte 129 and next 6 bits in the low end of byte 130 and so on. The above screenshot indicates that all the calculations are correct, ie where it should get the bit and where it should put it. However the code actually doing the getting and putting is commented out:
Code:
'-----------------------------------------------------------------------------------
' Buffer.0[Putbit] = Temp
' HSEROUT[" Reading back: ", DEC Buffer.0[Putbit],13]
'-----------------------------------------------------------------------------------
If I uncomment these two lines everything breaks. Not only does it not work but it destorys the original data in the low end of array:
Like I said, I've stared at this for most part of the day and I just don't understand what's going on. Does anyone see anything weird, strange or even better something that's just wrong?
Thank you in advance!
/Henrik.
Bookmarks